Haunted House
Team Project
OVERVIEW
TOOLS
Visual Studio 2022
I've created this project as a part of Computer Graphics module. It's a simple game but we had to program all of the stages from programmable rendering pipeline in order to correctly display our graphics with all of the system we wanted to implement. Thanks to this project I gained a lot of knowledge on how does PRP works as all of the modern engines has those built in with only changeable details. In this game you wake up in a house that is locked and you need to find a key in order to escape it. We have used DirectX api.
DirectX
LANGUAGE
C++
MEDIA GALLERY
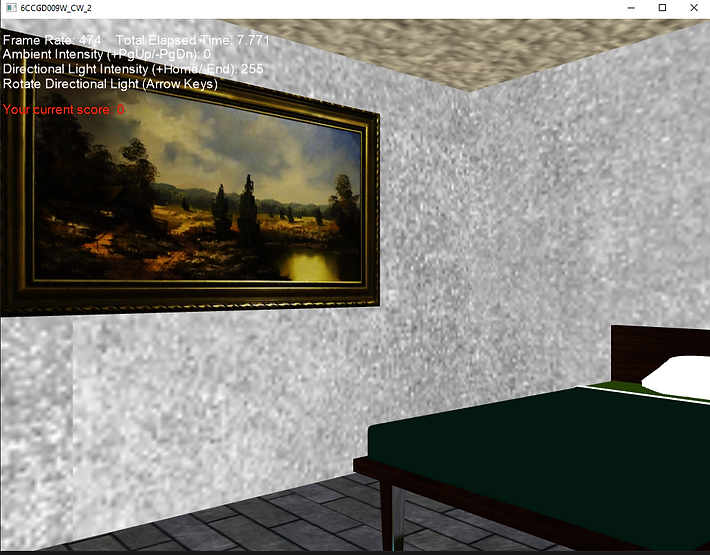.png)
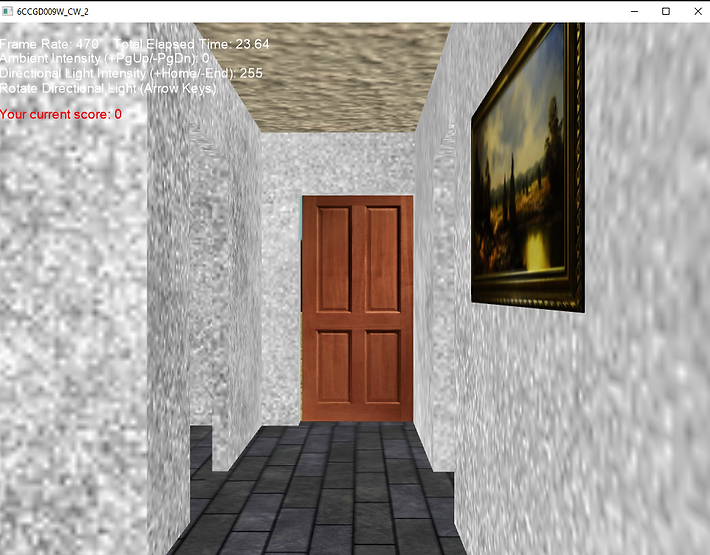.png)
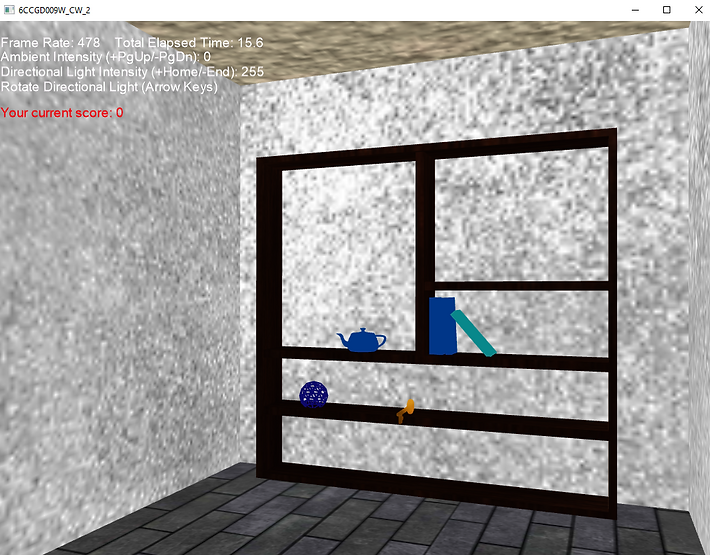.png)
SAMPLE CODE
Pick up mechanic
XMFLOAT4X4 P;
XMStoreFloat4x4(&P, mCamera->ProjectionMatrix());
//Compute picking ray in view space.
float vx = (+2.0f * sx / Game::DefaultScreenWidth - 1.0f) / P(0, 0);
float vy = (-2.0f * sy / Game::DefaultScreenHeight + 1.0f) / P(1, 1);
// Ray definition in view space.
XMVECTOR rayOrigin = XMVectorSet(0.0f, 0.0f, 0.0f, 1.0f);
XMVECTOR rayDir = XMVectorSet(vx, vy, -1.0f, 0.0f);
// Tranform ray to local space of Mesh via the inverse of both of view and world transform
XMMATRIX V = mCamera->ViewMatrix();
XMVECTOR vDeterminant = XMMatrixDeterminant(V);
XMMATRIX invView = XMMatrixInverse(&vDeterminant, V);
XMMATRIX W = XMLoadFloat4x4(model->WorldMatrix());
XMVECTOR vWorld = XMMatrixDeterminant(W);
XMMATRIX invWorld = XMMatrixInverse(&vWorld, W);
XMMATRIX toLocal = XMMatrixMultiply(invView, invWorld);
rayOrigin = XMVector3TransformCoord(rayOrigin, toLocal);
rayDir = XMVector3TransformNormal(rayDir, toLocal);
// Make the ray direction unit length for the intersection tests.
rayDir = XMVector3Normalize(rayDir);
float tmin = 0.0;
if (model->mBoundingBox.Intersects(rayOrigin, rayDir, tmin))
{
std::wostringstream pickupString;
pickupString << L"You found the Key to the door. It is now open: " <<'\n' << '\t' << '+' << model->ModelValue() << L" points";
int result = MessageBox(0, pickupString.str().c_str(), L"Object Found", MB_ICONASTERISK | MB_YESNO);
if (result == IDYES)
{
//hide the object
model->SetVisible(false);
//update the score
mScore += model->ModelValue();
}
}