Text Game
Solo Project
OVERVIEW
TOOLS
Visual Studio 2021
I've created this project as a part of Game programming patterns module. Our task was to create a console based game. We had to allow player to choose which path he wants to go and explore. The biggest issue was to make this game load all of the locations and items from text file without any specific pattern.
LANGUAGE
C++
MEDIA GALLERY
.png)
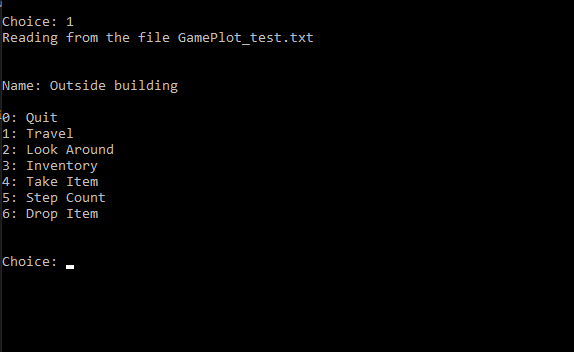

SAMPLE CODE
Inventory cpp
#include "Inventory.h"
Inventory::Inventory()
{
this->cap = 100;
this->nrOfItems = 0;
this->itemArr = new Item * [cap];
}
Inventory::~Inventory()
{
for (size_t i = 0; i < nrOfItems; i++)
{
delete this->itemArr[i];
}
delete[] itemArr;
}
void Inventory::initialize(const int from)
{
for (size_t i = 0; i < cap; i++)
{
this->itemArr[i] = nullptr;
}
}
void Inventory::expand()
{
this->cap *= 2;
Item** tempArr = new Item*[this->cap];
for (size_t i = 0; i < this->nrOfItems; i++)
{
tempArr[i] = new Item(*this->itemArr[i]);
}
for (size_t i = 0; i < this->nrOfItems; i++)
{
delete this->itemArr[i];
}
delete[] this->itemArr;
this->itemArr = tempArr;
this->initialize(this->nrOfItems);
}
void Inventory::addItem(const Item& item)
{
if (this->nrOfItems >= this->cap)
{
expand();
}
this->itemArr[this->nrOfItems++] = new Item(item);
}
void Inventory::removeItem(int index)
{
itemArr[index] = new Item;
}