WROUGHT
Team Project
OVERVIEW
Welcome to the steampunk era… Welcome to the world of Pirates!
Wrought is a 2D RPG game where the player will be playing the role of a pirate. You wake as the main character on an unknown island with no recollection of how you got to this place. The character realizes quickly that he doesn’t have any memories at all. The main goal is going to be to find out your history to somehow bring back your forgotten memories.
On your way to the final goal, you will encounter many challenges. You will encounter many different creatures and characters. Some of them will help you on your quest and some will do everything in their power to make your life harder. You will not only be able to forge your future but also to forge your tools to do so. We want to create a game where you will be able to craft your weapons with many different combinations.
​
We want to make Wrought easy to learn and intuitive for players. There will be a lot of interesting and diverse tasks to play through, as well as a splendid plot.
TOOLS
Monogame
LANGUAGE
C#
ROLE
Team Leader
Lead Programmer
MEDIA GALLERY
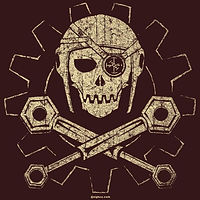
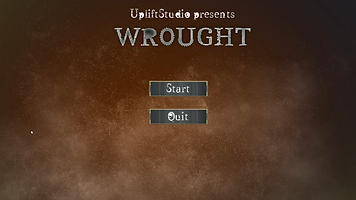.png)
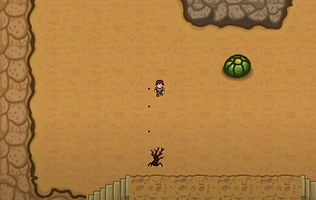.png)
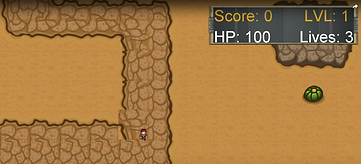.png)
SAMPLE CODE
Projectile class
using System;
using System.Collections.Generic;
using System.Text;
using Microsoft.Xna.Framework;
using Microsoft.Xna.Framework.Graphics;
using Microsoft.Xna.Framework.Input;
using WroughtDemo.Classes;
using Comora;
namespace WroughtDemo
{
class Projectile
{
#region Declarations for Projectiles
public static List<Projectile> projectiles = new List<Projectile>();
private Vector2 position;
private int speed = 400;
public int radius = 18;
private Dir direction;
private bool collided = false;
public int damage;
#endregion
#region Properties
public bool Collided
{
get { return collided; }
set { collided = value; }
}
public Projectile(Vector2 newPos, Dir newDir)
{
position = newPos;
direction = newDir;
}
public Vector2 Position
{
get { return position; }
}
#endregion
#region Method Call
public void Update(GameTime gameTime)
{
float dt = (float)gameTime.ElapsedGameTime.TotalSeconds;
switch (direction)
{
case Dir.Down:
position.Y += speed * dt;
break;
case Dir.Up:
position.Y -= speed * dt;
break;
case Dir.Left:
position.X -= speed * dt;
break;
case Dir.Right:
position.X += speed * dt;
break;
default:
break;
}
}
#endregion
}
}